The LCD 4004 for the Arduino IDE
On this page I want to show how to use a 40 characters 4 rows display with my Noiasca Liquid Crystal library.
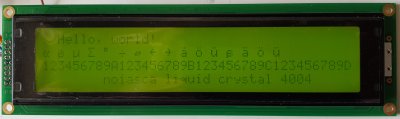
These 4004 LC displays basically consist of two 4002 displays. Therefore they have two enable lines and you will need an additional pin to drive the second enable line. As the library needs to know which logical driver should be used, the library must track which line you are currently using. Depending on the line either IC 1 or IC2 will be activated.
As always you have to include the library and the respective hardware implementation:
#include <NoiascaLiquidCrystal.h> #include <NoiascaHW/lcd_4bit.h> // parallel interface, 4bit
The constructor will need a lot of parameters, so it is a good idea to define some constants:
const byte cols = 40; // 40 columns/characters per row (must be correct for the 40x4) const byte rows = 4; // 4 rows const byte rs = 8; // RS pin const byte en = 9; // enable pin for first IC (upper rows) const byte en2 = 11; // enable pin for second IC (lower rows) const byte d4 = 4; // data pin const byte d5 = 5; // data pin const byte d6 = 6; // data pin const byte d7 = 7; // data pin const byte bl = 255; // set to 255 if not used const t_backlightPol blType = POSITIVE; // set to either POSITIVE, NEGATIVE
At the time of the implementation the RW pin is not used. But don't forget to connect the RW pin to GND! If you leave this LCD pin floating, it's very likely that the display isn't showing anything at all.
The type of backlight defines if the backlight pin is HIGH active or LOW active. Currently only POSITIVE is supported.
When you have defined all parameters you can create your LCD object:
LiquidCrystal_4bit_4004 lcd(rs, en, en2, d4, d5, d6, d7, bl, blType, cols, rows);
By default, some special characters and the German Umlauts will be printed accordingly. If you want to use another character converter, you can add a callback function:
LiquidCrystal_4bit_4004 lcd(rs, en, en2, d4, d5, d6, d7, bl, blType, cols, rows, convert_small); // a converter (Ä gets ä)
If you want to learn more about character converting, you should read here.

Caveats
Not all possible hardware interfaces are prepared for the 4004 display. Currently only the 4bit parallel interface and the PCF8574 expander will supports the 40 x 4 LCD. This is due to the additional needed pin. The typical 8channel expander has enough IOs for two enable lines and the backlight. You just have to rewire IO 1 (which might be used for RW) to Enable2 and connect the displays RW to GND.
Use this includes and constructor in case of the PCF8574:
#include <Wire.h> #include <NoiascaLiquidCrystal.h> #include <NoiascaHW/lcd_PCF8574.h> LiquidCrystal_PCF8574_4004 lcd(addr, rs, en, en2, d4, d5, d6, d7, bl, blType, cols, rows);
Summary
With the additional language converters it becomes very easy to print UTF8 characters on the 40 x 4 LCD.